python tutorial
Python For Loops
Learn more about Python, one of the world’s most versatile and popular programming languages.
Tutorial Contents
Loops are very useful programming principles for executing certain logic or a block of code multiple times for a collection of items like those found in string, list, tuple and dictionary data types.
One such looping technique is using a for
loop. A for loop is the most widely used looping technique for iterating over a list, dictionary, string or a tuple. It repeats a certain logic over and over again for different items unless there are no more items left or an exit-condition is met. An exit-condition is usually something that will stop the loop.
Let’s look at a small flow chart that explains how for loop works:
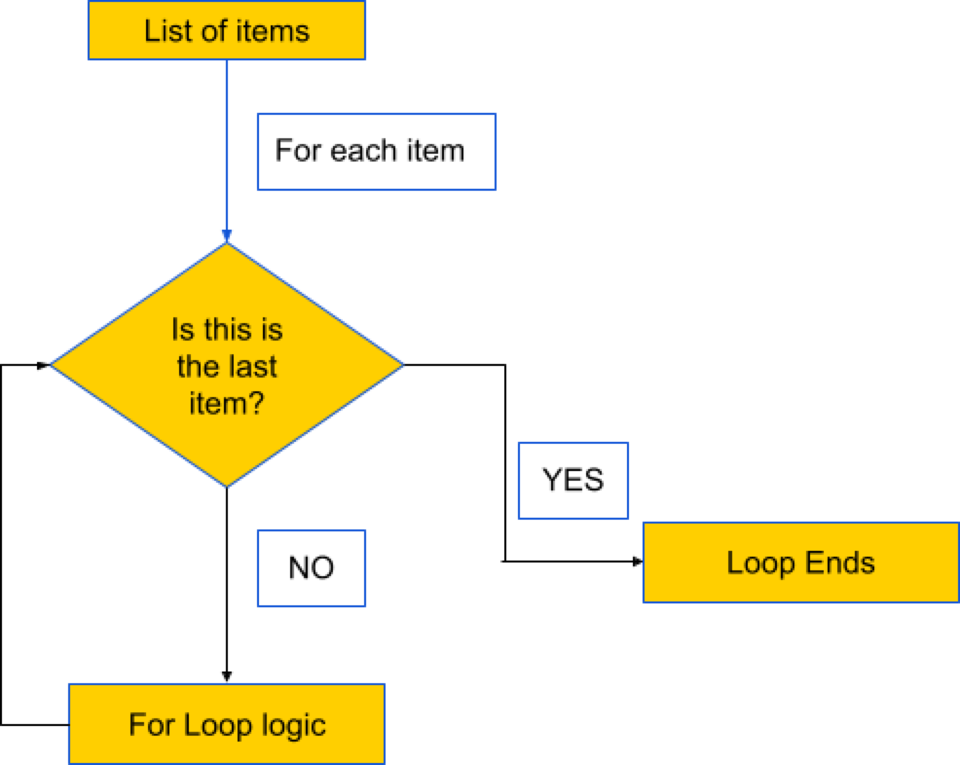
For loop in Python has following syntax:
for value in list:
for loop logic for value
Let’s look at how to use for loop in code snippet below:
>>> greeting = "hello"
>>> james_cameron_movies = ["Titanic", "Avatar", "The Terminator", "Aliens"]
>>> for character in greeting:
… print(character)
…
h
e
l
l
o
>>> for movie in james_cameron_movies:
… print(movie)
…
Titanic
Avatar
The Terminator
Aliens
Python Range Function
Sometimes while using a loop, you are not necessarily looping through a predefined list or string. Instead, you may wish to loop through a predefined range of values or numbers. range()
function comes really handy when you want to loop for a certain range of numbers. Based on the parameters passed to the range()
function, it will automatically generate those values, which can be then used inside of a for loop.
range()
function is defined as follows in two different variations:
range(stop)
range(start, stop[, step])
where, start
defines the starting number from which range should start. stop
defines the number at which the range should stop. The range will stop at the value defined by stop
minus 1. step
defines how the numbers should step up or increment in the range.
Let’s take a look at how range()
function might come handy inside a for loop syntax:
# print odd and even numbers between 1 to 10
# range starts at 1,
# ends at stopping point 11 - 1 which is 10
# step is 1 which means each time range value will be incremented by 1
>>> for num in range(1, 11, 1):
… if num % 2 == 0:
… print('Num ', num, ' is even')
… elif num % 2 != 0:
… print('Num ', num, ' is odd')
Num 1 is odd
Num 2 is even
Num 3 is odd
Num 4 is even
Num 5 is odd
Num 6 is even
Num 7 is odd
Num 8 is even
Num 9 is odd
Num 10 is even
# print all the numbers up to 5.
# when only one value is specified, it is the stop value
>>> for num in range(6):
… print(num)
…
0
1
2
3
4
5
Python Enumerate Function
When using loops, it is often handy for developers to remember the index or count of the item that has already been looped through. enumerate()
function in Python allows you to loop through an iterable while also providing the index or count of each item being looped through.
enumerate()
function is of the following form:
enumerate(iterable, start=0)
Let’s take a look at the code snippet below to understand how to use enumerate()
function with a for loop:
>>> greeting = "hello"
>>> james_cameron_movies = ["Titanic", "Avatar", "The Terminator", "Aliens"]
>>> for index, character in enumerate(greeting):
… print(character, ' is located at index ', index, ' in string ', greeting)
…
h is located at index 0 in string hello
e is located at index 1 in string hello
l is located at index 2 in string hello
l is located at index 3 in string hello
o is located at index 4 in string hello
# looping through the list using enumerate with start position as 1
>>> for index, movie in enumerate(james_cameron_movies, 1):
… print(movie, ' is located at index ', index, ' in list ', james_cameron_movies)
Titanic is located at index 1 in list ['Titanic', 'Avatar', 'The Terminator', 'Aliens']
Avatar is located at index 2 in list ['Titanic', 'Avatar', 'The Terminator', 'Aliens']
The Terminator is located at index 3 in list ['Titanic', 'Avatar', 'The Terminator', 'Aliens']
Aliens is located at index 4 in list ['Titanic', 'Avatar', 'The Terminator', 'Aliens']
Learn Python Today
Get hands-on experience writing code with interactive tutorials in our free online learning platform.
- Free and fun
- Designed for beginners
- No downloads or setup required